Metamap button
What is Metamap button
Metamap button is a web component that allows customers to start verification process from your page.
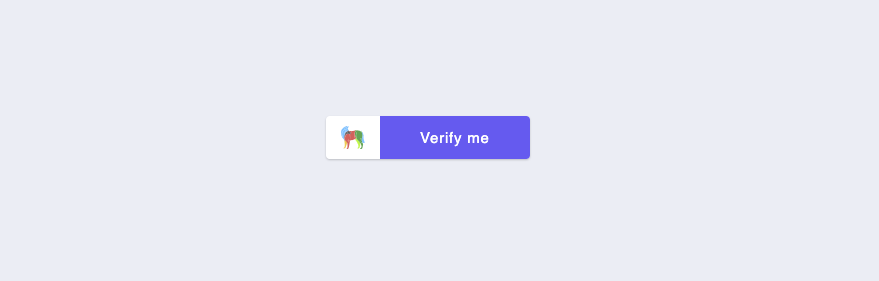
When should you use Metamap button
You should use Metamap button if you have existing web page from which you want your customers to start verification process.
Importing scripts
In order to use Metamap button on your page you will need to add button.js
to your page like so
<script src="https://web-button.metamap.com/button.js"></script>
Add Metamap button element
<metamap-button
clientid="your_client_id"
flowid="your_flow_id"
></metamap-button>
Replace your_client_id
and your_flow_id
with ids from your dashboard.
Learn how to obtain client and flow ids here.
Configuration
Configuration of Metamap button is done via html attributes. You can find complete list of attributes here
Interacting with Metamap button
During verification process Metamap button emits custom events that indicate certain state of verification process.
This event can be listened to by adding event listeners to button element
<metamap-button
clientid="your_client_id"
flowid="your_flow_id"
></metamap-button>
<script>
const metamapButton = document.querySelector('metamap-button');
metamapButton.addEventListener('metamap:userFinishedSdk', ({ detail }) => console.log(detail));
</script>
Events
Event name | Description | Payload |
---|---|---|
metamap:loaded | Indicates that verification scripts are loaded. Emitted before verification itself starts. | n/a |
metamap:userStartedSdk | Indicates that the individual has started verification process. Emitted just after verification was created. | identityId, verificationId |
metamap:userFinishedSdk | Indicates that the individual finished verification process. Emitted when the individual reaches final screen. | identityId, verificationId |
metamap:exitedSdk | Indicates that the individual exited verification before finishing it. Emitted when the individual chooses to exit verification. | identityId, verificationId |
metamap:continuedOnAnotherDevice | Indicates that the individual chose to continue redirection on another device. Emitted when individual chooses redirection method. | identityId verificationId |
metamap:screen | Indicates that the individual reached to an specific screen. Emitted when individual lands on the screen. | identityId verificationId screen |
Screen Names
This is meant to be a guide for you to use as potential names you could see in the MetaMap button metamap:screen
event.
Screen names are subject to change.
[
"verificationStart",
"verificationExit",
"verificationExitAskReasons",
"verificationExpired",
"verificationSuccess",
"verificationBackToDesktop",
"languageSelect",
"documentsOverview",
"documentCountrySelect",
"DocumentRegionSelect",
"documentSelect",
"documentHint",
"proofOfResidencyHint",
"documentCamera",
"documentCaptureIncode",
"documentPreview",
"documentUpload",
"documentUploading",
"emailInput",
"emailEnterCode",
"selfieHint",
"selfieCamera",
"selfiePreview",
"selfieUpload",
"selfieCaptureIncode",
"livenessHint",
"livenessFallbackHint",
"livenessCamera",
"livenessPreview",
"livenessFallbackPreview",
"livenessUpload",
"voicelivenessHint",
"voicelivenessCamera",
"VoicelivenessPreview",
"voicelivenessUpload",
"uploadError",
"uploadSuccess",
"eSignatureDocuments",
"proofOfOwnershipHint",
"proofOfOwnershipCamera",
"proofOfOwnershipPreview",
"redirectVerification",
"redirectEmailWaiting",
"redirectEmailForm",
"redirectQRCodeWaiting",
"redirectQRCodeInstructions",
"redirectGalleryDenied",
"phoneEnter",
"phoneCountrySelect",
"phoneSmsEnterCode",
"redirectBiometricFallback",
"permissionsInstructions",
"loading",
"documentSubtypeSelect",
"unsupportedBrowsers",
"maxFileSizeError",
"minFileSizeError",
"cameraDeniedError",
"inputAccessError",
"documentFaceDetectError",
"biometryFaceDetectError",
"documentNotAllDataError",
"DocumentMRZError",
"DocumentMismatchError",
"commonError",
"livenessMovementError",
"livenessVoiceError",
"noTextError",
"timeoutError",
"ipRestrictions",
"IncorrectSideError",
"DynamicInput",
"VideoAggrement",
"documentOcclusionError",
"RetriesBlockedError"
]
enum Screens {
VerificationStart = 'verificationStart',
VerificationExit = 'verificationExit',
VerificationExitAskReasons = 'verificationExitAskReasons',
VerificationExpired = 'verificationExpired',
VerificationSuccess = 'verificationSuccess',
VerificationBackToDesktop = 'verificationBackToDesktop',
LanguageSelect = 'languageSelect',
DocumentsOverview = 'documentsOverview',
DocumentCountrySelect = 'documentCountrySelect',
DocumentRegionSelect = 'DocumentRegionSelect',
DocumentSelect = 'documentSelect',
DocumentHint = 'documentHint',
ProofOfResidencyHint = 'proofOfResidencyHint',
DocumentCamera = 'documentCamera',
DocumentCaptureIncode = 'documentCaptureIncode',
DocumentPreview = 'documentPreview',
DocumentUpload = 'documentUpload',
DocumentUploading = 'documentUploading',
EmailEnter = 'emailInput',
EmailEnterCode = 'emailEnterCode',
SelfieHint = 'selfieHint',
SelfieCamera = 'selfieCamera',
SelfiePreview = 'selfiePreview',
SelfieUpload = 'selfieUpload',
SelfieCaptureIncode = 'selfieCaptureIncode',
LivenessHint = 'livenessHint',
LivenessFallbackHint = 'livenessFallbackHint',
LivenessCamera = 'livenessCamera',
LivenessPreview = 'livenessPreview',
LivenessFallbackPreview = 'livenessFallbackPreview',
LivenessUpload = 'livenessUpload',
VoicelivenessHint = 'voicelivenessHint',
VoicelivenessCamera = 'voicelivenessCamera',
VoicelivenessPreview = 'VoicelivenessPreview',
VoicelivenessUpload = 'voicelivenessUpload',
UploadError = 'uploadError',
UploadSuccess = 'uploadSuccess',
ESignatureDocuments = 'eSignatureDocuments',
ProofOfOwnershipHint = 'proofOfOwnershipHint',
ProofOfOwnershipCamera = 'proofOfOwnershipCamera',
ProofOfOwnershipPreview = 'proofOfOwnershipPreview',
RedirectVerification = 'redirectVerification',
RedirectEmailWaiting = 'redirectEmailWaiting',
RedirectEmailForm = 'redirectEmailForm',
RedirectQRCodeWaiting = 'redirectQRCodeWaiting',
RedirectQRCodeInstructions = 'redirectQRCodeInstructions',
RedirectGalleryDenied = 'redirectGalleryDenied',
PhoneEnter = 'phoneEnter',
PhoneCountrySelect = 'phoneCountrySelect',
PhoneSmsEnterCode = 'phoneSmsEnterCode',
RedirectBiometricFallback = 'redirectBiometricFallback',
PermissionsInstructions = 'permissionsInstructions',
Loading = 'loading',
DocumentSubtypeSelect = 'documentSubtypeSelect',
UnsupportedBrowsers = 'unsupportedBrowsers',
MaxFileSizeError = 'maxFileSizeError',
MinFileSizeError = 'minFileSizeError',
CameraDeniedError = 'cameraDeniedError',
InputAccessError = 'inputAccessError',
DocumentFaceDetectError = 'documentFaceDetectError',
BiometryFaceDetectError = 'biometryFaceDetectError',
DocumentNotAllDataError = 'documentNotAllDataError',
DocumentMRZError = 'DocumentMRZError',
DocumentMismatchError = 'DocumentMismatchError',
CommonError = 'commonError',
LivenessMovementError = 'livenessMovementError',
LivenessVoiceError = 'livenessVoiceError',
NoTextError = 'noTextError',
TimeoutError = 'timeoutError',
IpRestrictions = 'ipRestrictions',
IncorrectSideError = 'IncorrectSideError',
DynamicInput = 'DynamicInput',
VideoAggrement = 'VideoAggrement',
DocumentOcclusionError = 'documentOcclusionError',
RetriesBlockedError = 'RetriesBlockedError'
}
Updated 10 months ago